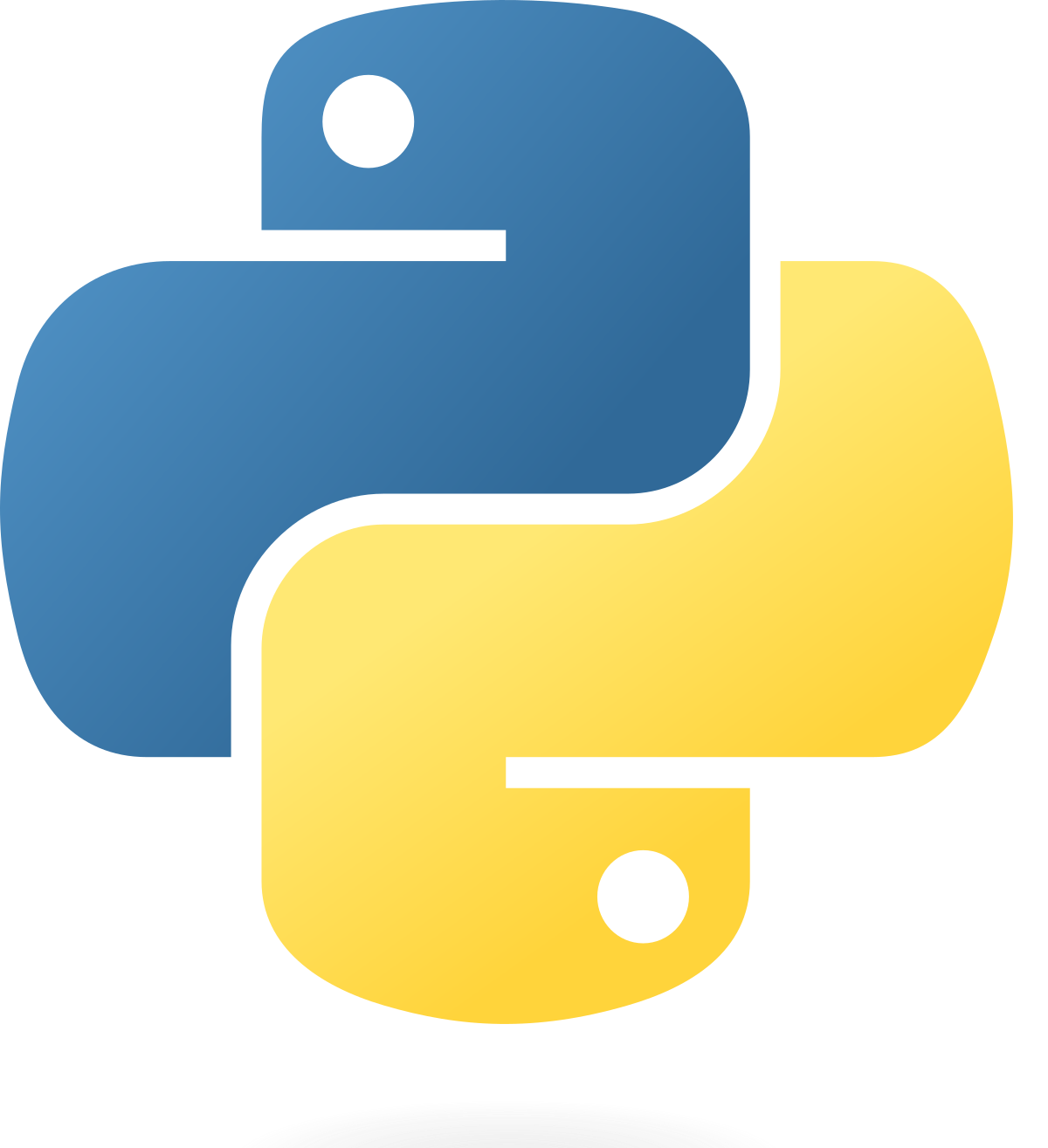
from collections import namedtuple
Person = namedtuple('Person', ['name', 'age', 'website'])
nash = Person('Nash', 43, 'https://nashs.website.com')
You can do ‘dot access’ instead of key access. It looks smart way compare with dictonary.
print(nash.name) # returns "Nash"
You can inherit Person namedtuple like below.
class Engineeer(Person):
def is_engineer(self):
if len(self.website) > 0:
return True
else:
return False
erica = Person('Erica', 31, '')
print(erica.is_engineer()) # False
NamedTuple can be used from typing. It looks smarter.
from typing import NamedTuple
class Person(NamedTuple):
name: str
age: int
website: str
John = Person('John', 33, 'https://johns.website.com')
It is useful for checking type.
>> print(type(John))
>> <class '__main__.Person'>