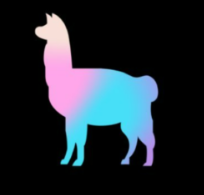
Load storage_context from Redis
from llama_index.storage.docstore import RedisDocumentStore
from llama_index.storage.index_store import RedisIndexStore
from llama_index.vector_stores import RedisVectorStore
from llama_index.storage.storage_context import StorageContext
from config import ConfigRedis
def get_storage_context(
cfg: ConfigRedis,
collection_id: str
) -> StorageContext:
docstore = RedisDocumentStore.from_host_and_port(
host=cfg.redis_host,
port=cfg.redis_port,
# namespace="llama_index"
)
index_store=RedisIndexStore.from_host_and_port(
host=cfg.redis_host,
port=cfg.redis_port,
# namespace="llama_index"
)
vector_store = RedisVectorStore(
index_name=collection_id,
# index_prefix="llama",
redis_url=f"redis://{cfg.redis_host}:{str(cfg.redis_port)}",
overwrite=True,
)
storage_context = StorageContext.from_defaults(
docstore=docstore,
index_store=index_store,
vector_store=vector_store
)
return storage_context
Config is here
@dataclass
class ConfigRedis():
redis_host: str
redis_port: int
@classmethod
def from_defaults(
cls,
redis_host: str,
redis_port: int
) -> 'ConfigRedis':
return cls(
redis_host,
redis_port
)
The easiest way to launch Redis is here.
docker run --name redis-vecdb -d -p 6379:6379 -p 8001:8001 redis/redis-stack:latest
Load llama-index from storage_context
from llama_index import load_index_from_storage
index = load_index_from_storage(storage_context)