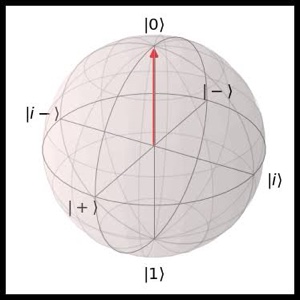
Phase values of Unitary Matrix
In quantum computing, each quantum gate is represented by a unitary matrix, and the entire quantum circuit is represented by the product of these matrices. However, manually calculating the product of these unitary matrices is a complex and time-consuming task. Especially as the number of qubits increases, the size of the matrices grows exponentially, making the calculations extremely difficult.
The unitary_simulator backend of Qiskit efficiently performs this calculation and provides the complete unitary matrix corresponding to the quantum circuit. The simulator automatically calculates the matrix representation of quantum gates and multiplies them appropriately to generate the overall unitary representation of the quantum circuit.
Furthermore, after generating the quantum circuit and producing the unitary matrix, the eigenvalues and phases of this unitary matrix are obtained. Since the eigenvalues are represented as complex numbers, the phase can be determined by calculating the exponent of the exponential.
Code
qc_unitary = QuantumCircuit(4)
# Apply Quantum Gate randomly
for qubit in range(4):
qc_unitary.rx(np.random.rand() * np.pi, qubit)
qc_unitary.ry(np.random.rand() * np.pi, qubit)
qc_unitary.rz(np.random.rand() * np.pi, qubit)
backend = Aer.get_backend('unitary_simulator')
unitary = qiskit.transpile(unitary_matrix, backend)
result = backend.run(unitary).result()
unitary_matrix_ = result.get_unitary(unitary)
numpy_matrix = np.array(unitary_matrix_)
eigenvalues, eigenvectors = np.linalg.eig(numpy_matrix)
phases = np.angle(eigenvalues)
The purpose of phase estimation is that to find theses phases using quantum circut.
Let’s call it a day for now.