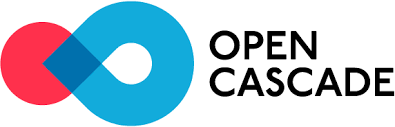
About
OpenCascade Technology is one of the few open-source options available for handling CAD data. It is written in C++ and is said to be incredibly complex. Since life is short, I decided to try using OCCT’s Python API. However, the documentation isn’t exactly great, so I’ve been experimenting while asking ChatGPT for help.
Code
I’ve started by analyzing CAD data, beginning with counting elements such as Faces, Shells, and Edges. Additionally, I’m checking whether the data is watertight or not.
from OCC.Core.TopoDS import TopoDS_Solid
from OCC.Core.TopExp import TopExp_Explorer
from OCC.Core.TopAbs import TopAbs_SHELL, TopAbs_FACE, TopAbs_EDGE, TopAbs_VERTEX, TopAbs_WIRE, TopAbs_SOLID
from OCC.Core.BRep import BRep_Tool
from OCC.Core.ShapeAnalysis import ShapeAnalysis_Shell
from OCC.Extend.DataExchange import read_step_file
def count_shapes(solid):
counts = {
"solids": 0,
"shells": 0,
"faces": 0,
"edges": 0,
"vertices": 0,
"wires": 0,
}
# Count solids
explorer = TopExp_Explorer(solid, TopAbs_SOLID)
while explorer.More():
counts["solids"] += 1
explorer.Next()
# Count shells
explorer = TopExp_Explorer(solid, TopAbs_SHELL)
while explorer.More():
counts["shells"] += 1
explorer.Next()
# Count faces
explorer = TopExp_Explorer(solid, TopAbs_FACE)
while explorer.More():
counts["faces"] += 1
explorer.Next()
# Count edges
explorer = TopExp_Explorer(solid, TopAbs_EDGE)
while explorer.More():
counts["edges"] += 1
explorer.Next()
# Count vertices
explorer = TopExp_Explorer(solid, TopAbs_VERTEX)
while explorer.More():
counts["vertices"] += 1
explorer.Next()
# Count wires
explorer = TopExp_Explorer(solid, TopAbs_WIRE)
while explorer.More():
counts["wires"] += 1
explorer.Next()
return counts
def is_watertight(solid):
"""
Check if the solid is watertight.
"""
explorer = TopExp_Explorer(solid, TopAbs_SHELL)
while explorer.More():
shell = explorer.Current()
shape_analysis = ShapeAnalysis_Shell()
if not shape_analysis.CheckClosed(shell):
return False # The shell is not closed
explorer.Next()
return True