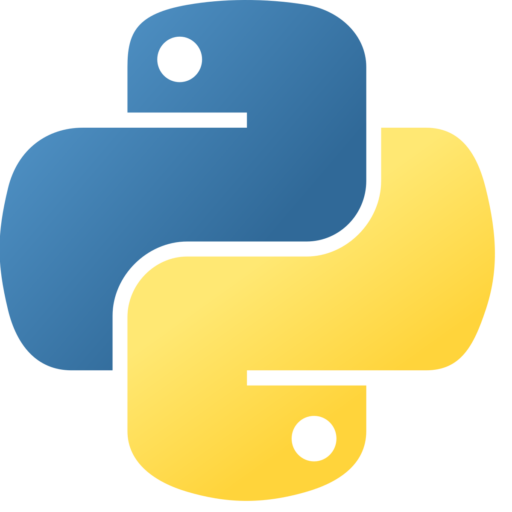
About
When you are going to set variables to BaseModel in Pydantic, you might want to validate the type of variables, or value itself.
Code Snippet
from pydantic import BaseModel, validator
class Person(BaseModel):
name: str
age: int
@validator("age", pre=True)
def convert_age(cls, value):
if isinstance(value, str):
try:
value = int(value)
except ValueError:
raise ValueError("Age must be an integer or a string that can be converted to an integer")
return value
@validator("age")
def check_age_range(cls, value):
if value > 105:
raise ValueError("you must be already dead")
if value < 0:
raise ValueError("you have not born yet")
return value
For sure we can constrain the range of variables with Feild, but validator is more flexible.
from pydantic import BaseModel, Field
class Person(BaseModel):
name: str
age: int = Field(..., ge=0, le=105)