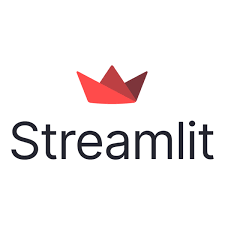
About
Streamlit is an excellent library for easily building UIs with Python. It offers more than enough features for creating small-scale applications. Additionally, Pydantic is a highly useful library for Python, a dynamically typed language. Since it allows for precise definitions and validation of value ranges, it is beneficial not only for use with Streamlit but also in many other scenarios. Therefore, I am noting down some commonly used design patterns that combine these two libraries. While there appears to be a library called streamlit-pydantic
, it currently does not work well due to version compatibility issues. Especially for the usage of slidebars and selectbox with Pydantic BaseModel.
Implementation
Sliders with Fields in BaseModel
import streamlit as st
from pydantic import BaseModel, Field
from annotated_types import Ge, Le
class Config(BaseModel):
v1 = Field(3, ge=3, le=10)
v2 = Field(1.0, ge=0.0, le=20.0)
v3 = Field(10, ge=0, le=100)
sliders = dict()
for name, info in Config.__fields__.items():
min_value = None
max_value = None
for ob in info.metadata:
if isinstance(ob, Ge):
min_value = ob.ge
if isinstance(ob, Le):
max_value = ob.le
default_value = info.default
sliders[name] = st.slider(
label=name,
min_value=min_value,
max_value=max_value,
value=default_value
)
SelectBox with Literal in BaseModel
from typing import Literal
class Config(BaseModel):
data: Literal["option1", "option2", "option3"]
data = Config.__annotations__['data'].__args__
data_selected = st.selectbox('box:', data)