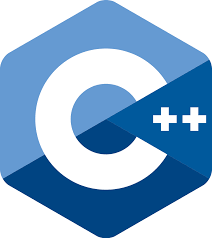
About
There some libraries to read json file format using C++ such as nlohmann/json, RapidJson, JsonCpp. I tested nlohmann/json.
Code
#include <CLI/CLI.hpp>
#include <iostream>
#include <fstream>
#include <nlohmann/json.hpp>
using json = nlohmann::json;
int main(int argc, char** argv) {
CLI::App app("Example CLI");
std::string filename;
app.add_option("-f,--filename", filename, "JSON file")->required();
CLI11_PARSE(app, argc, argv);
std::cout << "Filename: " << filename << std::endl;
std::ifstream json_file(filename);
if (!json_file) {
std::cerr << "could not open the file: " << filename << std::endl;
return 1;
}
json jsonData;
json_file >> jsonData;
std::cout << "Json Data: " << jsonData.dump(4) << std::endl;
if (jsonData.contains("name")) {
std::string name = jsonData["name"];
std::cout << "name: " << name << std::endl;
}
return 0;
}