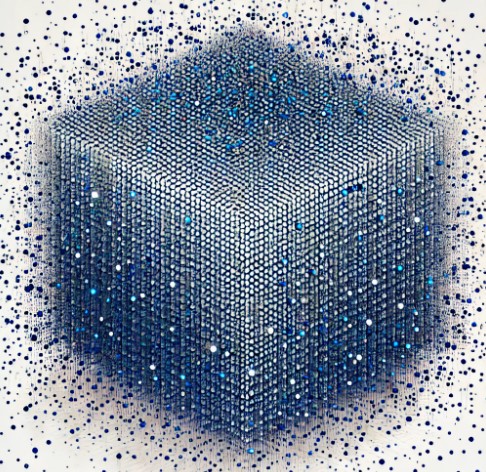
About
Under Construction
https://docs.scipy.org/doc/scipy/reference/sparse.html
- csc_array: Compressed Sparse Column format
- csr_array: Compressed Sparse Row format
- bsr_array: Block Sparse Row format
- lil_array: List of Lists format
- dok_array: Dictionary of Keys format
- coo_array: COOrdinate format (aka IJV, triplet format)
- dia_array: DIAgonal format
The document says that
To construct an array efficiently, use any of coo_array, dok_array or lil_array. dok_array and lil_array support basic slicing and fancy indexing with a similar syntax to NumPy arrays. The COO format does not support indexing (yet) but can also be used to efficiently construct arrays using coord and value info.
Despite their similarity to NumPy arrays, it is strongly discouraged to use NumPy functions directly on these arrays because NumPy typically treats them as generic Python objects rather than arrays, leading to unexpected (and incorrect) results. If you do want to apply a NumPy function to these arrays, first check if SciPy has its own implementation for the given sparse array class, or convert the sparse array to a NumPy array (e.g., using the toarray method of the class) before applying the method.
All conversions among the CSR, CSC, and COO formats are efficient, linear-time operations.
To perform manipulations such as multiplication or inversion, first convert the array to either CSC or CSR format. The lil_array format is row-based, so conversion to CSR is efficient, whereas conversion to CSC is less so.
Code Snippet
Block Matrix
N = 5000
A = sp.random(N, N, density=0.01, format='csr')
B = sp.random(N, N, density=0.01, format='csr')
C = sp.random(N, N, density=0.01, format='csr')
D = sp.random(N, N, density=0.01, format='csr')
block_matrix = sp.bmat([[A, B], [C, D]], format='csr')
print(block_matrix.shape) # (10000, 10000)
Solve Linear Equation with lsmr
lsmr solves the system of linear equations Ax = b. If the system is inconsistent, it solves the least-squares problem min ||b - Ax||^2.
import numpy as np
from scipy.sparse import csc_matrix
from scipy.sparse.linalg import lsmr
A = csc_matrix([[3, 0, 2],
[2, 0, -2],
[0, 1, 1]], dtype=float)
b = np.array([1, 2, 3], dtype=float)
x = lsmr(A, b)[0]
lil to csr/csc
# Good for intuitive understanding for human
A = sp.sparse.lil_matrix((m, n))
for r, c, v in zip(rows, cols, values):
A[r, c] = v
# better to convert to csr/csc for computation
A = A.tocsr()