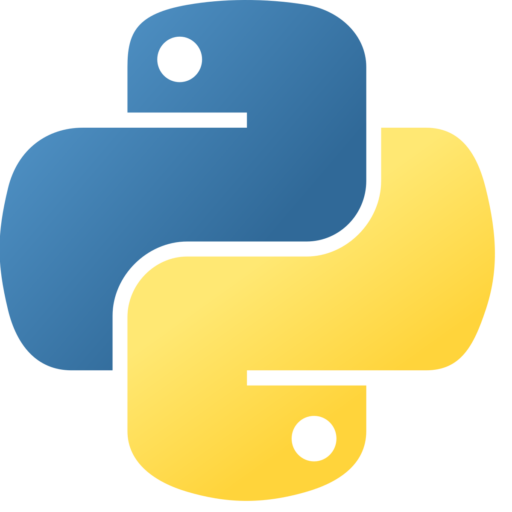
About
Python’s multipledispatch is a library for dynamically dispatching (selecting) functions based on multiple argument types, allowing you to define functions with the same name for multiple types and automatically switch between them.
Installation
pip install multipledispatch
Usage
Register functions using Decorator
from multipledispatch import dispatch
# a function recieve int type
@dispatch(int)
def greet(value):
print(f"Hello, you passed an integer: {value}")
# a function recieve str type
@dispatch(str)
def greet(value):
print(f"Hello, you passed a string: '{value}'")
greet(1234) # 出力: Hello, you passed an integer: 1234
greet("Hello World") # 出力: Hello, you passed a string: 'Hello World'
Register several functions using Dispatcher Object from multpledispatch
from multipledispatch import Dispatcher
area_calculator = Dispatcher("area_calculator")
# register function with types
@area_calculator.register(int, int)
def rectangle_area(width, height):
return width * height
@area_calculator.register(float)
def circle_area(radius):
import math
return math.pi * radius ** 2
# calling
print(area_calculator(4, 5))
print(area_calculator(3.0))