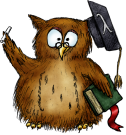
About
I wanted to try Eigen again after a long time, so I created a CMakeLists file and set up an environment to run it. Eigen is a C++ matrix operation library that operates very efficiently. It will be useful for various projects.
CmakeLists.txt
cmake_minimum_required(VERSION 3.14)
project(EigenExample)
set(CMAKE_CXX_STANDARD 17)
set(CMAKE_CXX_STANDARD_REQUIRED ON)
include(FetchContent)
FetchContent_Declare(
CLI11
GIT_REPOSITORY https://github.com/CLIUtils/CLI11.git
GIT_TAG v2.3.2
)
FetchContent_MakeAvailable(CLI11)
FetchContent_Declare(
Eigen3
GIT_REPOSITORY https://gitlab.com/libeigen/eigen.git
GIT_TAG 3.4.0
)
FetchContent_MakeAvailable(Eigen3)
add_executable(EigenExample src/main.cpp)
target_link_libraries(EigenExample PRIVATE CLI11 Eigen3::Eigen)
Small Test on Eigen
#include <iostream>
#include <CLI/CLI.hpp>
#include <Eigen/Dense>
int main(int argc, char** argv){
CLI::App app("Example CLI");
double e11 = 1.0;
double e12 = 2.0;
double e21 = 3.0;
double e22 = 4.0;
app.add_option("-a,--element11", e11, "Set e11");
app.add_option("-b,--element12", e12, "Set e12");
app.add_option("-c,--element21", e21, "Set e21");
app.add_option("-d,--element22", e22, "Set e22");
CLI11_PARSE(app, argc, argv);
Eigen::Matrix2d A;
A << double(e12), double(e12),
double(e21), double(e22);
Eigen::Vector2d b(5, 6);
Eigen::Vector2d x = A * b;
std::cout << "A:\n" << A << "\n";
std::cout << "b:\n" << b << "\n";
std::cout << "A * b:\n" << x << "\n";
Eigen::Matrix2d A_inv = A.inverse();
std::cout << "A inverted:\n" << A_inv << "\n";
return 0;
}
Build
cmake ..
cmake --build .
Built target CLI11
Consolidate compiler generated dependencies of target EigenExample
Building CXX object CMakeFiles/EigenExample.dir/src/main.cpp.o
Okay, seems that I stood at a point to start with it.