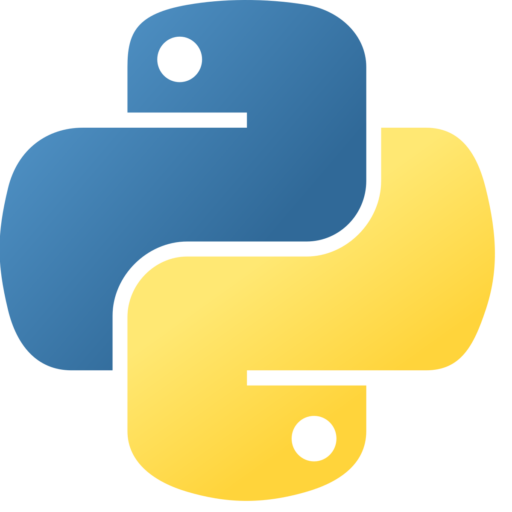
Saving memory
Specifying slots causes the instance’s attribute values to be stored in the form of an array of slots rather than a dictionary, reducing the processing time required to create the dictionary object and the amount of memory used. On the other hand, referencing an attribute in an instance with slots will slow down the process by several percent.
@dataclass(slots=True)
class Person:
name: str
age: int
address: str
Get variables as dictionary
from dataclasses import dataclass, asdict
@dataclass
class Person:
name: str
age: int
address: str
@classmethod
def from_defaults(
cls,
name: str,
age: int,
address: str
) -> 'Person':
return cls(
name, age, address
)
p = Person("Alice", 30, "Tokyo")
d = asdict(p)
print(d)
# {'name': 'Alice', 'age': 30, 'address': 'Tokyo'}